Workspace access and permissions
Before setting up the Slack notifier itself, you have to configure Slack as a 'chat client' for AWS Chatbot via the console, which gives it access to your Slack workspace. Luckily, this is a one-time process. Make sure you choose the correct workspace!
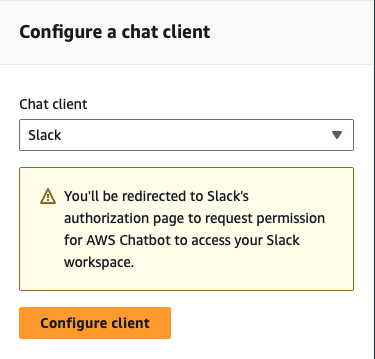
Slack notifier CDK configuration
While the notifier code can be directly defined in an individual CDK stack, for any multi-stack application (or if you need to send notifications to multiple Slack channels) it makes sense to define it as its own CDK construct that can be imported into any and all stacks.
The code below is an example of such a construct, with a few things worth considering:
- The
SlackNotifier
class exposes the SNS topic as an instance variable withself.topic
. This allows the topic to be specified as the target of a CloudWatch alarm action. - As explained in the AWS Chatbot docs, the actions that users can take from within the Slack channel are restricted by the "intersection of your guardrail policies and what is allowed by their roles". In the example below, the guardrail policy is a service-agnostic
ReadOnlyAccess
, with the channel role allowingCloudwatchReadOnlyAccess
. As the former is a superset of the latter, users will in effect be able to execute all actions allowed by theCloudwatchReadOnlyAccess
policy.
1 | |
2 | |
3 | |
4 | |
5 | |
6 | |
7 | |
8 | |
9 | |
10 | |
11 | |
12 | |
13 | |
14 | |
15 | |
16 | |
17 | |
18 | |
19 | |
20 | |
21 | |
22 | |
23 | |
24 | |
25 | |
26 | |
27 | |
Adding CloudWatch alarms
With the SlackNotifier
construct defined, we can configure CloudWatch alarms to push to the Slack channel. The code below is a simple demonstration of what can be achieved:
- The
SlackNotifier
is created with the relevant Slack credentials. - We then create the actual alarm. In this case we monitor when the number of messages in a SQS dead letter queue rises above 5.
- Lastly, the notifier SNS topic is configured as the target for the
OK
andALARM
CloudWatch actions so that a Slack notification is created when the CloudWatch metric both enters and exits theALARM
state.
1 | |
2 | |
3 | |
4 | |
5 | |
6 | |
7 | |
8 | |
9 | |
10 | |
11 | |
12 | |
13 | |
14 | |
15 | |
16 | |
17 | |
18 | |
19 | |
20 | |
Conclusion
Observability and monitoring systems are crucial components in modern web applications and services, providing real-time insights into performance issues as well as error detection and notification. In this blog post we've shown how CloudWatch alarms can be propagated to Slack, enabling prompt detection of potential issues in an application.
At Weird Sheep Labs, we've implemented systems such as this for multiple clients, helping them maintain better situational awareness about their applications and enabling them to respond to failures in a timely manner.
If this sounds like something your business could benefit from, please don't hesitate to get in touch!